Shapes
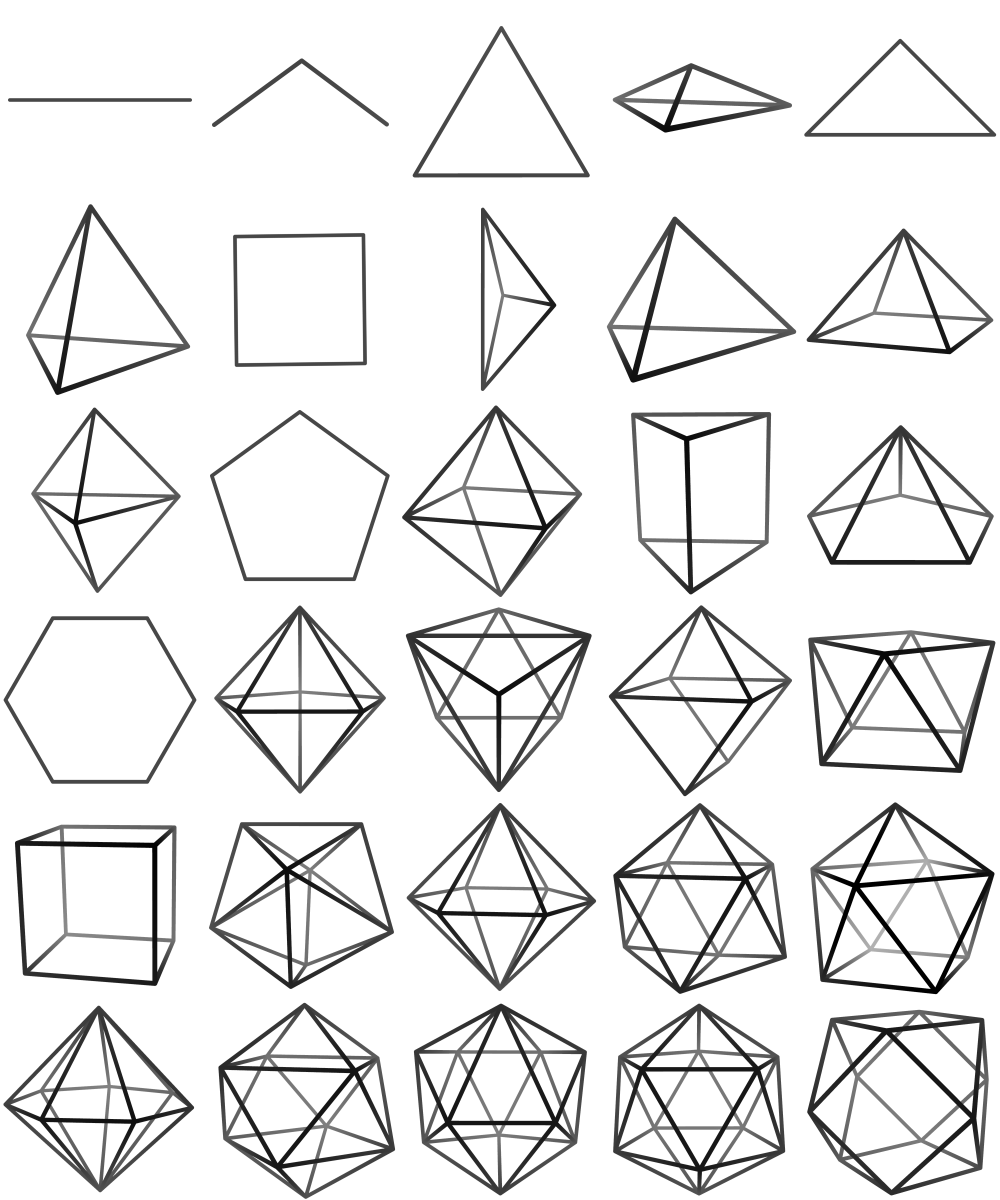
All shapes that molassembler can classify from positions and treat permutationally. These are shapes from 2 vertices (line, bent) up to 12 vertices (icosahedron, cuboctahedron).
Shapes are classified into molecules by determining the nearest shape with a matching number of vertices.
Shape submodule
- class scine_molassembler.shapes.Shape
Enumeration of recognizable polyhedral shapes
>>> all_shapes = shapes.Shape.__members__.values() # Full list of shapes >>> shapes.Shape.SquareAntiprism in all_shapes True >>> shapes.Shape.__members__["TrigonalPrism"] # String lookup by enum name <Shape.TrigonalPrism: 13> >>> str(shapes.Shape.TrigonalPrism) # displayable string 'trigonal prism'
Members:
Line
Bent
EquilateralTriangle
VacantTetrahedron
T
Tetrahedron
Square
Seesaw
TrigonalPyramid
SquarePyramid
TrigonalBipyramid
Pentagon
Octahedron
TrigonalPrism
PentagonalPyramid
Hexagon
PentagonalBipyramid
CappedOctahedron
CappedTrigonalPrism
SquareAntiprism
Cube
TrigonalDodecahedron
HexagonalBipyramid
TricappedTrigonalPrism
CappedSquareAntiprism
HeptagonalBipyramid
BicappedSquareAntiprism
EdgeContractedIcosahedron
Icosahedron
Cuboctahedron
- Bent = <Shape.Bent: 1>
- BicappedSquareAntiprism = <Shape.BicappedSquareAntiprism: 26>
- CappedOctahedron = <Shape.CappedOctahedron: 17>
- CappedSquareAntiprism = <Shape.CappedSquareAntiprism: 24>
- CappedTrigonalPrism = <Shape.CappedTrigonalPrism: 18>
- Cube = <Shape.Cube: 20>
- Cuboctahedron = <Shape.Cuboctahedron: 29>
- EdgeContractedIcosahedron = <Shape.EdgeContractedIcosahedron: 27>
- EquilateralTriangle = <Shape.EquilateralTriangle: 2>
- HeptagonalBipyramid = <Shape.HeptagonalBipyramid: 25>
- Hexagon = <Shape.Hexagon: 15>
- HexagonalBipyramid = <Shape.HexagonalBipyramid: 22>
- Icosahedron = <Shape.Icosahedron: 28>
- Line = <Shape.Line: 0>
- Octahedron = <Shape.Octahedron: 12>
- Pentagon = <Shape.Pentagon: 11>
- PentagonalBipyramid = <Shape.PentagonalBipyramid: 16>
- PentagonalPyramid = <Shape.PentagonalPyramid: 14>
- Seesaw = <Shape.Seesaw: 7>
- Square = <Shape.Square: 6>
- SquareAntiprism = <Shape.SquareAntiprism: 19>
- SquarePyramid = <Shape.SquarePyramid: 9>
- T = <Shape.T: 4>
- Tetrahedron = <Shape.Tetrahedron: 5>
- TricappedTrigonalPrism = <Shape.TricappedTrigonalPrism: 23>
- TrigonalBipyramid = <Shape.TrigonalBipyramid: 10>
- TrigonalDodecahedron = <Shape.TrigonalDodecahedron: 21>
- TrigonalPrism = <Shape.TrigonalPrism: 13>
- TrigonalPyramid = <Shape.TrigonalPyramid: 8>
- VacantTetrahedron = <Shape.VacantTetrahedron: 3>
- __init__(self: scine_molassembler.shapes.Shape, value: int) None
- name()
__str__(*args, **kwargs) Overloaded function.
__str__(self: scine_molassembler.shapes.Shape) -> str
__str__(self: handle) -> str
- property value
- scine_molassembler.shapes.coordinates(shape: scine_molassembler.shapes.Shape) numpy.ndarray[numpy.float64[3, n]]
Idealized spherical coordinates of the shape
- scine_molassembler.shapes.name_from_str(name_str: str) scine_molassembler.shapes.Shape
Fetch a shape name from its string representation. Case and whitespace-sensitive.
>>> s = shapes.Shape.CappedSquareAntiprism >>> str(s) 'capped square antiprism' >>> shapes.name_from_str(str(s)) == s True
- scine_molassembler.shapes.size(shape: scine_molassembler.shapes.Shape) int
Number of vertices of a shape. Does not include a centroid.
>>> shapes.size(shapes.Shape.Line) 2 >>> shapes.size(shapes.Shape.Octahedron) 6 >>> shapes.size(shapes.Shape.Cuboctahedron) 12